CI/CD Configuration for Different Providers
To run the CLI in a CI/CD pipeline we need to configure it to run at 2 different triggers:
- When a PR is opened or changed
- When a PR is merged
This is not supported in some providers - in that case we define the CLI to run whenever the default branch changes, and then we use the API to find a merged PR which contains the commit which was just pushed.
1. Generate a Swimm API keyβ
Navigate to your Swimm Workspace Settings and generate a Swimm API key.
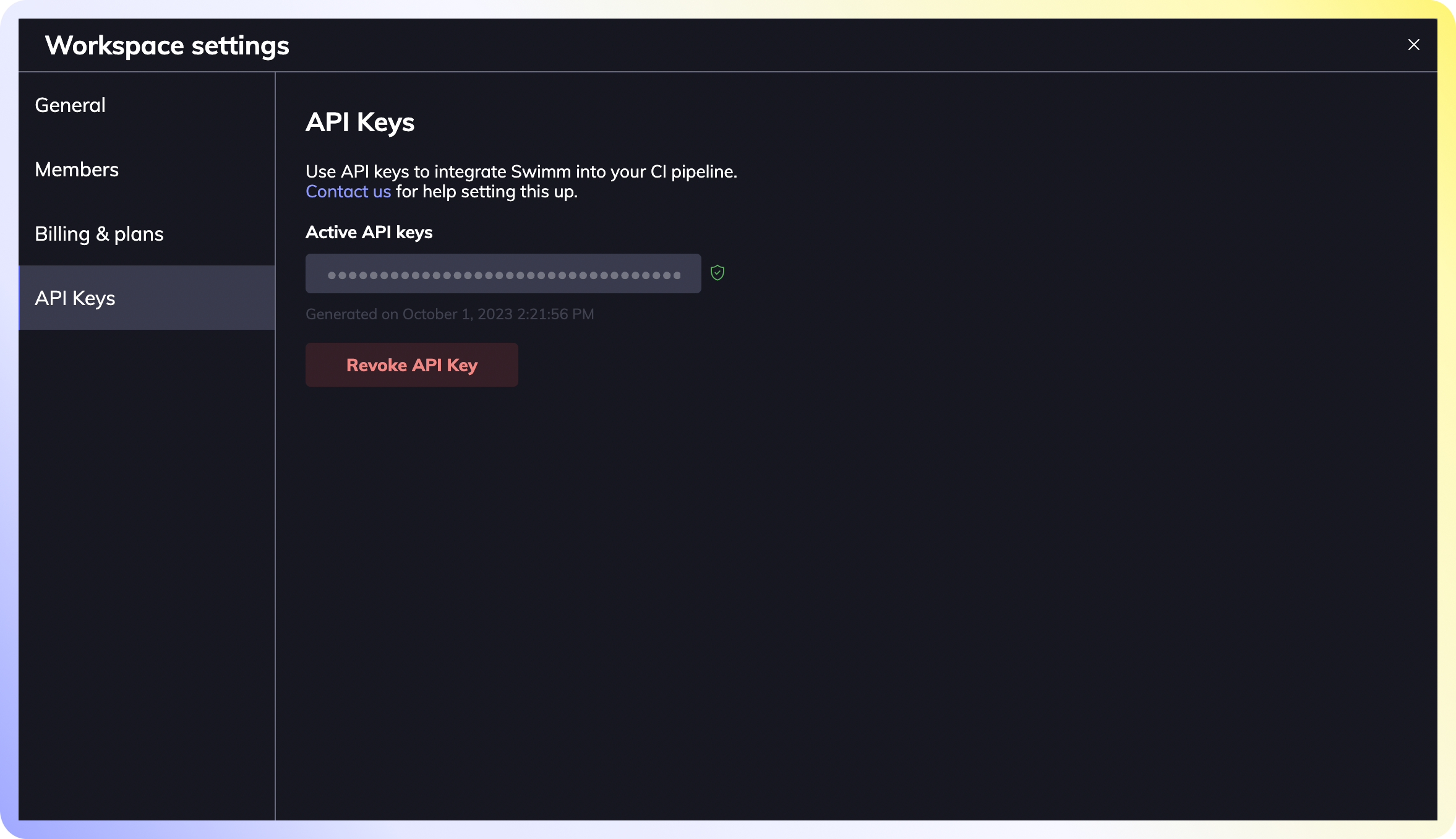
2. Configure your CI/CD providerβ
The following examples show how to configure this for each CI/CD provider. Note that this does not cover secret management.
GitHub (Enterprise)β
Create the .github/workflows/swm.yml
file and replace the relevant variables with the appropriate values.
name: SwimmCLI
on:
pull_request:
types: [opened, reopened, closed, edited, synchronize]
workflow_dispatch:
env:
SWIMM_API_KEY: swimm api key
GITHUB_TOKEN: private access token
ENTERPRISE_URL: the url to your GitHub server
GIT_CONFIG_NAME: a name for the Auto-sync commit
GIT_CONFIG_EMAIL: an email for the Auto-sync commit
permissions:
contents: write
pull-requests: write
jobs:
swimm:
runs-on: self-hosted
steps:
- name: Checkout Code
uses: actions/checkout@v2
with:
ref: ${{ github.event.pull_request.head.sha }}
fetch-depth: 0
persist-credentials: false
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: 18.x
- name: Install Dependencies
run: npm install @actions/core @actions/github
- name: Download and Setup Swimm CLI
run: |
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli
chmod +x ./swimm_cli
- name: Swimm Verification
if: github.event_name == 'pull_request' && github.event.action != 'closed'
env:
GITHUB_CONTEXT: ${{ toJson(github) }}
run: |
git config user.name $GIT_CONFIG_NAME
git config user.email $GIT_CONFIG_EMAIL
./swimm_cli verify --swimm-api-key $SWIMM_API_KEY --github ${{ secrets.GITHUB_TOKEN }} --github-params "$GITHUB_CONTEXT" --github-url $ENTERPRISE_URL --github $GITHUB_TOKEN 1>&2
- name: Execute On Merge
if: github.ref == 'refs/heads/main' && github.event.pull_request.merged == true
env:
GITHUB_CONTEXT: ${{ toJson(github) }}
run: |
./swimm_cli onmerge --swimm-api-key $SWIMM_API_KEY --github ${{ secrets.GITHUB_TOKEN }} --github-params "$GITHUB_CONTEXT" --github-url $ENTERPRISE_URL 1>&2
GitLab (Cloud and Enterprise)β
- Initialize Repo
- GitLab CI/CD
- Project Access Token
Initialize your repository with Swimm.
- A swimm.json file should exist in the default branch.
- If it doesn't exist, simply create your first Swimm document and it will be created for you.
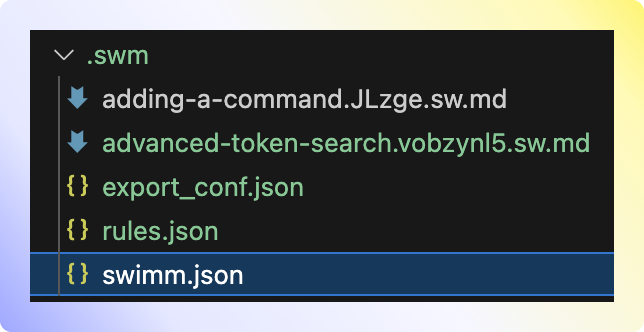
Under the repo settings (in GitLab), navigate to:
"General" -> "Visibility, project features, permissions" -> click "Expand."

Then, go to the Repository -> toggle "CI/CD."
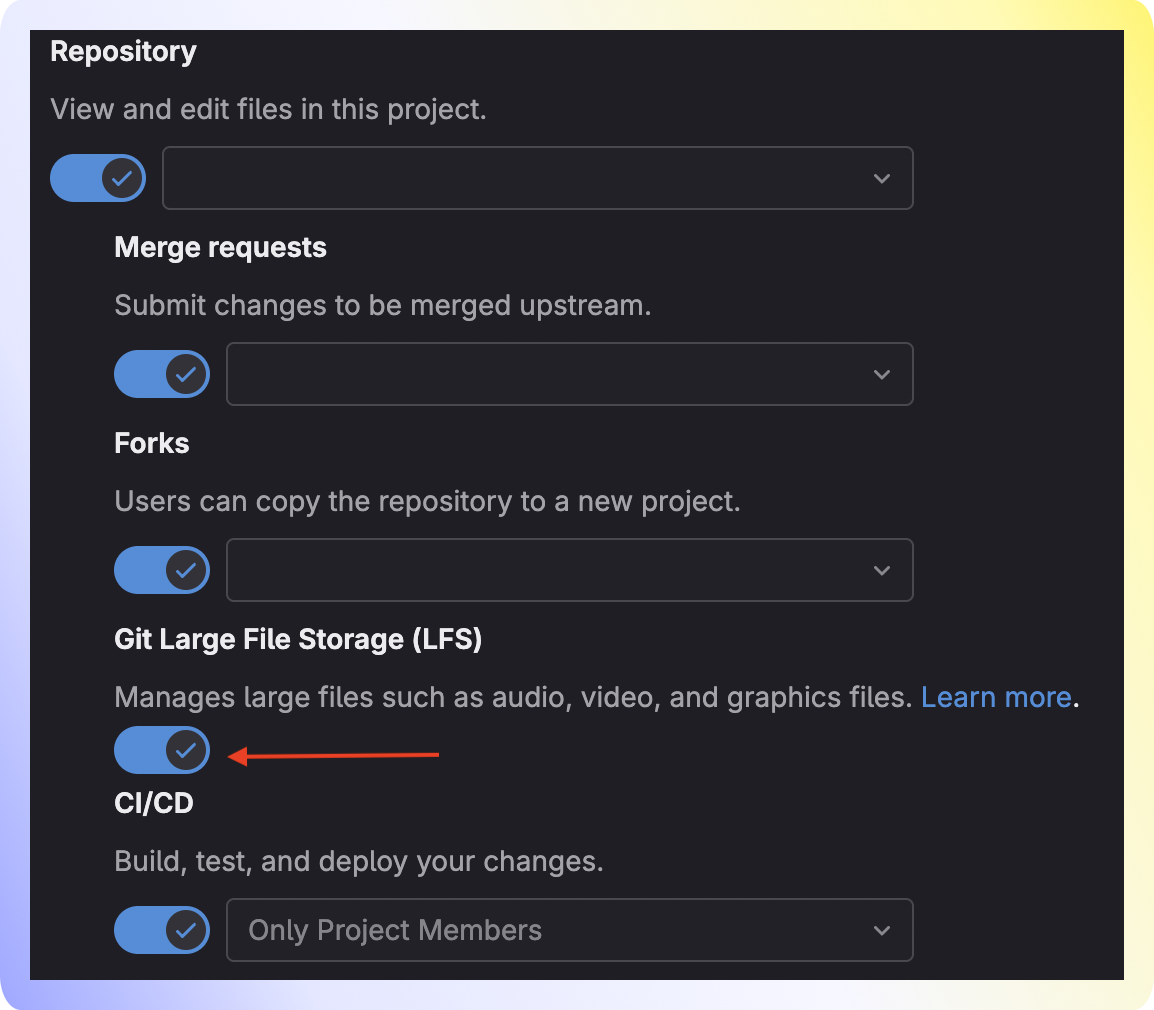
Under the repo settings:
- Create a Project Access Token.
- Navigate to Access Tokens.
- Create a token with the Developer role with all scopes enabled.
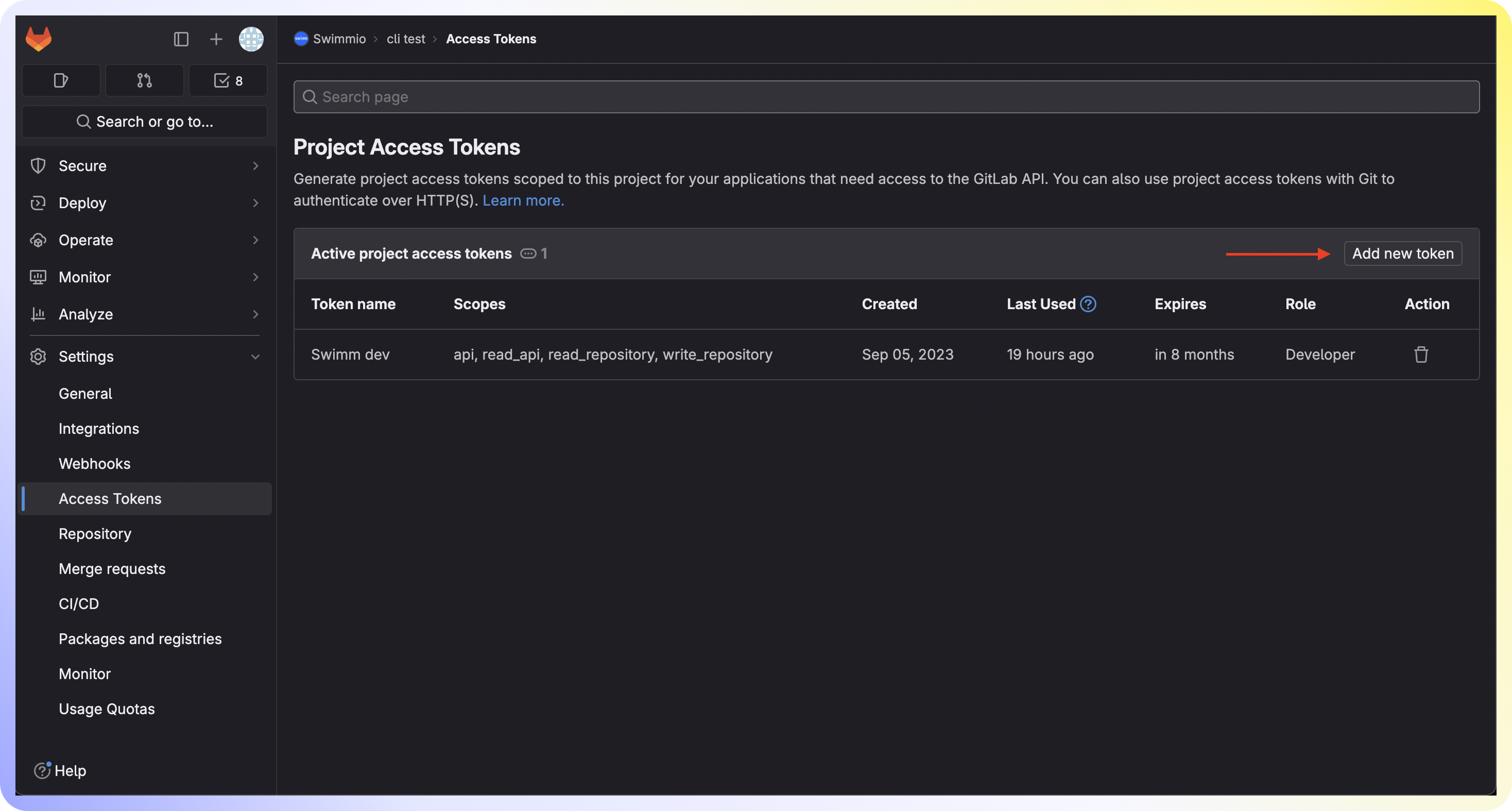
Create the .gitlab-ci.yml
file and replace the relevant variables with the appropriate values.
GitLab (Cloud):β
variables:
SWIMM_API_KEY: "swimm api key (located in workspace settings)"
GITLAB_TOKEN: "project/repo token"
GIT_CONFIG_NAME: "name for the Auto-sync commit"
GIT_CONFIG_EMAIL: "email for the Auto-sync commit"
merge_request:
image: node:latest
rules:
- if: $CI_PIPELINE_SOURCE == 'merge_request_event'
variables:
GIT_DEPTH: 1000
script:
- >
git config user.name $GIT_CONFIG_NAME && git config user.email $GIT_CONFIG_EMAIL &&
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli verify
--force-colors
--swimm-api-key $SWIMM_API_KEY
--gitlab $GITLAB_TOKEN
on_merge:
image: node:latest
rules:
- if: $CI_COMMIT_BRANCH == $CI_DEFAULT_BRANCH
variables:
GIT_DEPTH: 1000
script:
- git branch $CI_COMMIT_REF_NAME
- >
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli onmerge
--swimm-api-key $SWIMM_API_KEY
--gitlab $GITLAB_TOKEN
GitLab (Enterprise):β
variables:
SWIMM_API_KEY: "swimm api key (located in workspace settings)"
GITLAB_TOKEN: "project/repo token"
GITLAB_URL: "url to your GitLab server"
GIT_CONFIG_NAME: "name for the Auto-sync commit"
GIT_CONFIG_EMAIL: "email for the Auto-sync commit"
merge_request:
image: node:latest
rules:
- if: $CI_PIPELINE_SOURCE == 'merge_request_event'
variables:
GIT_DEPTH: 1000
tags: [docker]
script:
- >
git config user.name $GIT_CONFIG_NAME && git config user.email $GIT_CONFIG_EMAIL &&
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli verify
--force-colors
--swimm-api-key $SWIMM_API_KEY
--gitlab $GITLAB_TOKEN
--gitlab-url $GITLAB_URL
on_merge:
image: node:latest
rules:
- if: $CI_COMMIT_BRANCH == $CI_DEFAULT_BRANCH
variables:
GIT_DEPTH: 1000
tags: [docker]
script:
- >
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli onmerge
--swimm-api-key $SWIMM_API_KEY
--gitlab $GITLAB_TOKEN
--gitlab-url $GITLAB_URL
Bitbucket (Cloud)β
Note: Bitbucket Cloud does not support variables, so you will need to define these values in your configuration:
Values | Description |
---|---|
SWIMM_API_KEY | The API key from your Swimm workspace settings. |
BITBUCKET_ACCESS_TOKEN | The project access token. |
REPOS_DEFAULT_BRANCH | The default branch of your repository. |
USER_NAME_FOR_COMMIT | A name to be used for Auto-sync commits. |
USER_EMAIL_FOR_COMMIT | An email address for Auto-sync commits. |
Create the .bitbucket-pipelines.yml
file.
image: node:18
clone:
depth: 'full'
pipelines:
pull-requests:
'**':
- parallel:
- step:
name: Build and Test
caches:
- node
script:
- >
git config user.name USER_NAME_FOR_COMMIT && git config user.email USER_EMAIL_FOR_COMMIT &&
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli verify -k SWIMM_API_KEY --bitbucket BITBUCKET_ACCESS_TOKEN
branches:
REPOS_DEFAULT_BRANCH:
- step:
script:
- >
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli onmerge -k SWIMM_API_KEY --bitbucket BITBUCKET_ACCESS_TOKEN
Bitbucket DC (with Jenkins)β
- Our script is tailored for the Bitbucket Branch Source plugin. For setup instructions, please review its documentation.
- Contact us if you use another plugin, as additional testing and development are required to support different plugins.
Create the Jenkins
file (Groovy) and replace the relevant variables with the appropriate values.
pipeline {
agent any
environment {
SWIMM_API_KEY = 'swimm api key (located in workspace settings)'
BITBUCKET_TOKEN = 'project/repo token'
BITBUCKET_URL = 'url to your bitbucket server ending with /rest'
GIT_CONFIG_NAME = 'name for the Auto-sync commit'
GIT_CONFIG_EMAIL = 'email for the Auto-sync commit'
DEFAULT_BRANCH = 'the repositories default branch'
}
stages {
stage('On Merge') {
when {
branch "${env.DEFAULT_BRANCH}"
}
steps {
nodejs(nodeJSInstallationName: '18.17.1') {
sh '''
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli onmerge \
--swimm-api-key ${env.SWIMM_API_KEY} \
--bitbucket-dc-url ${env.BITBUCKET_URL} \
--bitbucketDc ${env.BITBUCKET_TOKEN}
'''
}
}
}
stage('Verify') {
when {
changeRequest()
}
steps {
nodejs(nodeJSInstallationName: '18.17.1') {
sh '''
git config user.name ${env.GIT_CONFIG_NAME} && git config user.email ${env.GIT_CONFIG_EMAIL} &&
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli &&
chmod +x ./swimm_cli &&
./swimm_cli verify \
--swimm-api-key ${env.SWIMM_API_KEY} \
--bitbucket-dc-url ${env.BITBUCKET_URL} \
--bitbucketDc ${env.BITBUCKET_TOKEN}
'''
}
}
}
}
}
Bambooβ
These scripts are designed for integrating Swimm verification with Bitbucket in a Bamboo CI/CD pipeline. Replace these placeholders in the following two scripts:
Placeholder | Description |
---|---|
YOUR_BITBUCKET_DC_SERVER_ADDRESS | Your Bitbucket instance address |
YOUR_BITBUCKET_DC_TOKEN | Your access token* |
*Ensure your access token has necessary permissions for your repository. |
Notesβ
- Add as an inline script in the Bamboo plan configuration.
- Use a "Shell" task for execution.
- This script will be executed on the Bamboo agent.
- Environment variables will not be defined if this script is run a "repo file."
Script: On-Push Verificationβ
This script triggers when a new update to a Pull Request (PR) occurs, and will run the Swimm CLI to verify changes in the PR.
# Define required environment parameters
export CHANGE_ID="${bamboo.repository.pr.key}"
export CHANGE_BRANCH=${bamboo.repository.pr.sourceBranch}
export GIT_COMMIT=${bamboo.repository.revision.number}
export GIT_URL=${bamboo.planRepository.repositoryUrl}
# Setup and run Swimm CLI
git config user.name swimm && git config user.email temp@swimm.io
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli
chmod +x ./swimm_cli
./swimm_cli --version
./swimm_cli verify --swimm-api-key SWIMM_API_KEY --bitbucket-dc-url YOUR_BITBUCKET_DC_SERVER_ADDRESS --bitbucketDc YOUR_BITBUCKET_DC_TOKEN
Script: On-Merge Verificationβ
This script executes the Swimm CLI to verify changes on your default branch after a PR is merged.*
# Define required environment parameters
export CHANGE_BRANCH=${bamboo.planRepository.branch}
export GIT_COMMIT=${bamboo.repository.revision.number}
export GIT_URL=${bamboo.planRepository.repositoryUrl}
# Setup and run Swimm CLI
wget -q -O swimm_cli https://releases.swimm.io/ci/latest/packed-swimm-linux-cli
chmod +x ./swimm_cli
./swimm_cli --version
./swimm_cli onmerge --swimm-api-key SWIMM_API_KEY --bitbucket-dc-url YOUR_BITBUCKET_DC_SERVER_ADDRESS --bitbucketDc YOUR_BITBUCKET_DC_TOKEN
*Assumes a full checkout of the repository is done beforehand.